Note
Go to the end to download the full example code.
Fluid Simulation Result Extraction#
This example shows how to load a fluid simulation (here a heating coil simulated with CFX), explore the metadata available, and use the result extraction capabilities.
Note
This example requires DPF 7.0 (2024.1.pre0) or above. For more information, see Compatibility.
Perform required imports#
from ansys.dpf import post
from ansys.dpf.post import examples
Load the fluid analysis result#
fluid_example_files = examples.download_cfx_heating_coil()
simulation = post.FluidSimulation(
cas=fluid_example_files["cas"], dat=fluid_example_files["dat"]
)
# Printing the simulation will show most of the available metadata
print(simulation)
Fluid Simulation.
Data Sources
------------------------------
DPF DataSources:
Result files:
result key: cas and path: /opt/hostedtoolcache/Python/3.10.15/x64/lib/python3.10/site-packages/ansys/dpf/core/examples/result_files/cfx-heating_coil/def.cas.cff
Secondary files:
path: /opt/hostedtoolcache/Python/3.10.15/x64/lib/python3.10/site-packages/ansys/dpf/core/examples/result_files/cfx-heating_coil/def.dat.cff
DPF Model
------------------------------
Static analysis
Unit system: SI: m, kg, N, s, V, A, K
Physics Type: Fluid
Available results:
- specific_heat: Nodal Specific Heat
- epsilon: Nodal Epsilon
- enthalpy: Nodal Enthalpy
- turbulent_kinetic_energy: Nodal Turbulent Kinetic Energy
- thermal_conductivity: Nodal Thermal Conductivity
- dynamic_viscosity: Nodal Dynamic Viscosity
- turbulent_viscosity: Nodal Turbulent Viscosity
- static_pressure: Nodal Static Pressure
- total_pressure: Nodal Total Pressure
- density: Nodal Density
- entropy: Nodal Entropy
- temperature: Nodal Temperature
- total_temperature: Nodal Total Temperature
- velocity: Nodal Velocity
Available qualifier labels:
- phase: <Mixture> (1), Water at 25 C (2), Copper (3)
- zone: outflow (9), Default (10), inflow (11), Solid 2.8 1 (12), Solid 2.7 1 (13), Solid 2.6 1 (14), Solid 2.5 1 (15), Solid 2.4 1 (16), Solid 2.3 1 (17), Default 1 (1), Default (18), Solid 2.3 (19), Solid 2.4 (20), Solid 2.5 (21), Solid 2.6 (22), Solid 2.7 (23), Solid 2.8 (24), heater (2)
------------------------------
DPF Meshed Region:
25456 nodes
74882 elements
Unit: m
With solid (3D) elements
------------------------------
DPF Time/Freq Support:
Number of sets: 1
Cumulative Frequency () LoadStep Substep
1 0.000000 1 1
Explore the available metadata#
Check the available cell and face zones
print(simulation.cell_zones)
print(simulation.face_zones)
{1: 'Default 1', 2: 'heater'}
{9: 'outflow', 10: 'Default', 11: 'inflow', 12: 'Solid 2.8 1', 13: 'Solid 2.7 1', 14: 'Solid 2.6 1', 15: 'Solid 2.5 1', 16: 'Solid 2.4 1', 17: 'Solid 2.3 1', 18: 'Default', 19: 'Solid 2.3', 20: 'Solid 2.4', 21: 'Solid 2.5', 22: 'Solid 2.6', 23: 'Solid 2.7', 24: 'Solid 2.8'}
The mesh metadata is available separately from the mesh as accessing the mesh means loading it. Use the mesh_info property to explore the mesh structure to define queries
print(simulation.mesh_info)
Fluid mesh metadata
-------------------
Number of nodes: 25456
Number of faces: 11780
Number of cells: 74882
Cell zones:
{1: 'Default 1', 2: 'heater'}
Face zones:
{9: 'outflow', 10: 'Default', 11: 'inflow', 12: 'Solid 2.8 1', 13: 'Solid 2.7 1', 14: 'Solid 2.6 1', 15: 'Solid 2.5 1', 16: 'Solid 2.4 1', 17: 'Solid 2.3 1', 18: 'Default', 19: 'Solid 2.3', 20: 'Solid 2.4', 21: 'Solid 2.5', 22: 'Solid 2.6', 23: 'Solid 2.7', 24: 'Solid 2.8'}
Cell to face zones:
{1: [9, 10, 11, 12, 13, 14, 15, 16, 17], 2: [18, 19, 20, 21, 22, 23, 24]}
Check the available species
print(simulation.species)
0 species available
{}
Check the available phases
print(simulation.phases)
3 phases available
{Phase<name: '<Mixture>', id=1>, Phase<name: 'Water at 25 C', id=2>, Phase<name: 'Copper', id=3>, }
- Extract a result
print(simulation.result_info)
Static analysis
Unit system: SI: m, kg, N, s, V, A, K
Physics Type: Fluid
Available results:
- specific_heat: Nodal Specific Heat
- epsilon: Nodal Epsilon
- enthalpy: Nodal Enthalpy
- turbulent_kinetic_energy: Nodal Turbulent Kinetic Energy
- thermal_conductivity: Nodal Thermal Conductivity
- dynamic_viscosity: Nodal Dynamic Viscosity
- turbulent_viscosity: Nodal Turbulent Viscosity
- static_pressure: Nodal Static Pressure
- total_pressure: Nodal Total Pressure
- density: Nodal Density
- entropy: Nodal Entropy
- temperature: Nodal Temperature
- total_temperature: Nodal Total Temperature
- velocity: Nodal Velocity
Available qualifier labels:
- phase: <Mixture> (1), Water at 25 C (2), Copper (3)
- zone: outflow (9), Default (10), inflow (11), Solid 2.8 1 (12), Solid 2.7 1 (13), Solid 2.6 1 (14), Solid 2.5 1 (15), Solid 2.4 1 (16), Solid 2.3 1 (17), Default 1 (1), Default (18), Solid 2.3 (19), Solid 2.4 (20), Solid 2.5 (21), Solid 2.6 (22), Solid 2.7 (23), Solid 2.8 (24), heater (2)
Print information about a specific available result
print(simulation.result_info["temperature"])
# Or use an index
# print(simulation.result_info[12])
DPF Result
----------
temperature
Operator name: "TEMP"
Number of components: 1
Dimensionality: scalar
Homogeneity: temperature
Units: K
Location: Nodal
Available qualifier labels:
- zone: Default 1 (1), outflow (9), Default (10), inflow (11), Solid 2.8 1 (12), Solid 2.7 1 (13), Solid 2.6 1 (14), Solid 2.5 1 (15), Solid 2.4 1 (16), Solid 2.3 1 (17), heater (2), Default (18), Solid 2.3 (19), Solid 2.4 (20), Solid 2.5 (21), Solid 2.6 (22), Solid 2.7 (23), Solid 2.8 (24)
- phase: Water at 25 C (2), Copper (3)
Available qualifier combinations:
{'zone': 1, 'phase': 2}
{'zone': 9, 'phase': 2}
{'zone': 10, 'phase': 2}
{'zone': 11, 'phase': 2}
{'zone': 12, 'phase': 2}
{'zone': 13, 'phase': 2}
{'zone': 14, 'phase': 2}
{'zone': 15, 'phase': 2}
{'zone': 16, 'phase': 2}
{'zone': 17, 'phase': 2}
{'zone': 2, 'phase': 3}
{'zone': 18, 'phase': 3}
{'zone': 19, 'phase': 3}
{'zone': 20, 'phase': 3}
{'zone': 21, 'phase': 3}
{'zone': 22, 'phase': 3}
{'zone': 23, 'phase': 3}
{'zone': 24, 'phase': 3}
Extract the temperature data#
temperature = simulation.temperature()
print(temperature)
# # The dataframe obtained shows data for two different phases
results TEMP (K)
set_ids 1
phase Water at 25 C (2) Copper (3)
node_ids
1 3.0533e+02
2 3.0389e+02
3 3.0155e+02
4 3.0201e+02
5 3.0340e+02
6 3.0487e+02
... ... ...
Select data for phase 2 only (Water)
print(temperature.select(phase=[2]))
results TEMP (K)
set_ids 1
phase Water at 25 C (2)
node_ids
1 3.0533e+02
2 3.0389e+02
3 3.0155e+02
4 3.0201e+02
5 3.0340e+02
6 3.0487e+02
... ...
To directly extract the temperature data for a given phase, pass the ‘temperature’ method a ‘phases’ argument. This argument must be given a list of phase unique identifiers, which appear in the dataframe in the phase label column between parentheses, or as listed under the ‘Available qualifier labels’ section of the metadata on the result You can also directly use the phase name.
water_temperature = simulation.temperature(phases=["Water at 25 C"])
# equivalent to
# water_temperature = simulation.temperature(phases=[2])
print(water_temperature)
# # The dataframe obtained now only stores data for the water phase.
results TEMP (K) ...
set_ids 1 ...
phase Water at 25 C (2) ...
zone Default 1 (1) outflow (9) Default (10) inflow (11) Solid 2.8 1 (12) Solid 2.7 1 (13) ...
node_ids ...
1 3.0533e+02 3.0533e+02 ...
2 3.0389e+02 3.0389e+02 ...
3 3.0155e+02 3.0155e+02 ...
4 3.0201e+02 3.0201e+02 ...
5 3.0340e+02 3.0340e+02 ...
6 3.0487e+02 3.0487e+02 ...
... ... ... ... ... ... ... ...
To extract a result on given zones use the ‘zone_ids’ argument or the ‘qualifiers’ dictionary argument with key ‘zone’ Here we request and plot the temperature on all face zones
face_temperature = simulation.temperature(zone_ids=list(simulation.face_zones.keys()))
face_temperature.plot()
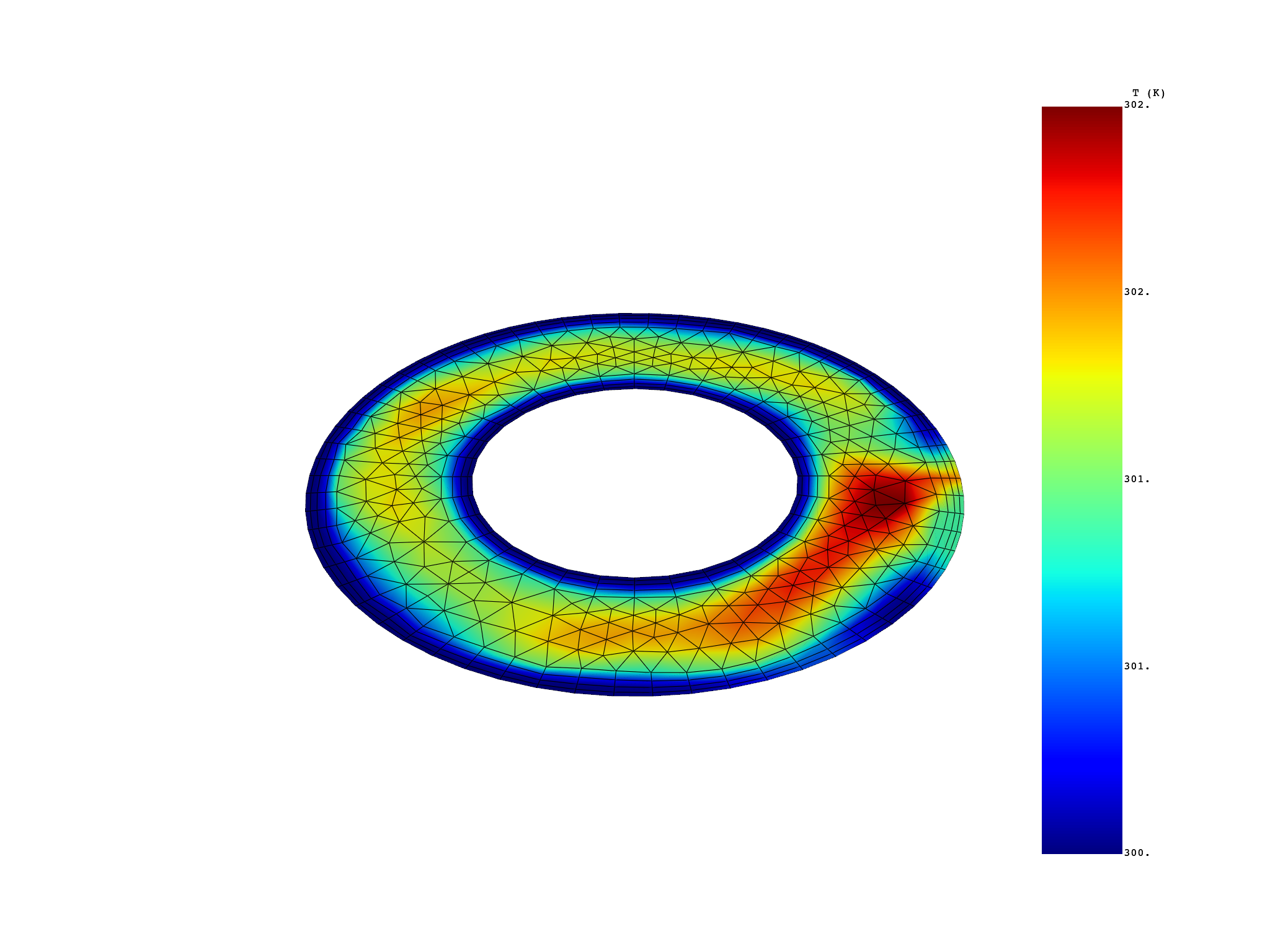
Total running time of the script: (0 minutes 3.293 seconds)