Note
Go to the end to download the full example code.
Static analysis#
This example shows how you can post-process a result file for a static analysis using PyDPF-Post.
Perform required imports#
Perform required imports.
from ansys.dpf import post
from ansys.dpf.post import examples
Get Solution
object#
Get the Solution
object. This example loads a result file for a static analysis
computed in Ansys Mechanical.
example_path = examples.download_all_kinds_of_complexity()
solution = post.load_solution(example_path)
print(solution)
Static Analysis Solution object.
Data Sources
------------------------------
DPF DataSources:
Result files:
result key: rst and path: /opt/hostedtoolcache/Python/3.10.15/x64/lib/python3.10/site-packages/ansys/dpf/core/examples/result_files/testing/allKindOfComplexity.rst
Secondary files:
DPF Model
------------------------------
Static analysis
Unit system: MKS: m, kg, N, s, V, A, degC
Physics Type: Mechanical
Available results:
- displacement: Nodal Displacement
- reaction_force: Nodal Force
- elemental_summable_miscellaneous_data: Elemental Elemental Summable Miscellaneous Data
- element_nodal_forces: ElementalNodal Element nodal Forces
- stress: ElementalNodal Stress
- elemental_volume: Elemental Volume
- stiffness_matrix_energy: Elemental Energy-stiffness matrix
- artificial_hourglass_energy: Elemental Hourglass Energy
- thermal_dissipation_energy: Elemental thermal dissipation energy
- kinetic_energy: Elemental Kinetic Energy
- co_energy: Elemental co-energy
- incremental_energy: Elemental incremental energy
- elastic_strain: ElementalNodal Strain
- thermal_strain: ElementalNodal Thermal Strains
- thermal_strains_eqv: ElementalNodal Thermal Strains eqv
- swelling_strains: ElementalNodal Swelling Strains
- element_euler_angles: ElementalNodal Element Euler Angles
- elemental_non_summable_miscellaneous_data: Elemental Elemental Non Summable Miscellaneous Data
- structural_temperature: ElementalNodal Structural temperature
- contact_status: ElementalNodal Contact Status
- contact_penetration: ElementalNodal Contact Penetration
- contact_pressure: ElementalNodal Contact Pressure
- contact_friction_stress: ElementalNodal Contact Friction Stress
- contact_total_stress: ElementalNodal Contact Total Stress
- contact_sliding_distance: ElementalNodal Contact Sliding Distance
- contact_gap_distance: ElementalNodal Contact Gap Distance
- total_heat_flux_at_contact_surface: ElementalNodal Total heat flux at contact surface
- contact_status_changes: ElementalNodal Contact status changes
- fluid_penetration_pressure: ElementalNodal Fluid Penetration Pressure
------------------------------
DPF Meshed Region:
15129 nodes
10294 elements
Unit: m
With solid (3D) elements, shell (2D) elements, shell (3D) elements, beam (1D) elements
------------------------------
DPF Time/Freq Support:
Number of sets: 1
Cumulative Time (s) LoadStep Substep
1 1.000000 1 1
Get Result
objects#
Get displacement result#
Get the displacement Result
object.
disp_result = solution.displacement()
disp = disp_result.vector
print(disp)
Displacement result.
This result has been computed using dpf.core.Operator objects, which
have been chained together according to the following list:
- U: Result operator. Compute the desired result.
Check number of fields#
Check the number of fields.
print(disp.num_fields)
1
Get data from field#
Get data from a field.
print(disp.get_data_at_field(0))
[[ 9.84182297e-06 5.13025031e-06 -6.66435651e-07]
[ 9.95996777e-06 4.93526360e-06 -6.25236961e-07]
[ 9.93154893e-06 5.88552090e-06 -7.83251832e-07]
...
[ 5.00000000e-03 -1.54556837e-04 0.00000000e+00]
[ 5.00000000e-03 -1.56813550e-04 0.00000000e+00]
[ 5.00000000e-03 -1.66125455e-04 0.00000000e+00]]
Get maximum data value over all fields#
Get the maximum data value over all fields.
print(disp.max_data)
[[8.50619058e+04 1.04659292e+01 3.73620870e+05]]
Get minimum data value over all fields#
Get the minimum data value over all fields.
print(disp.min_data)
[[-1.82645944e-06 -1.04473039e+01 -2.94677257e-04]]
Get maximum data value over targeted field#
Get the maximum data value over a targeted field.
print(disp.get_max_data_at_field(0))
[8.50619058e+04 1.04659292e+01 3.73620870e+05]
Get minimum data value over all fields#
Get the minimum data value over all fields.
print(disp.get_min_data_at_field(0))
[-1.82645944e-06 -1.04473039e+01 -2.94677257e-04]
Get stress result#
Get the stress Result
object for a tensor.
stress_result = solution.stress()
stress = stress_result.tensor
Check number of fields#
Check the number of shell and solid elements in distinct fields.
print(stress.num_fields)
2
Get shell field#
Get the shell field.
shell_field = stress[0]
print(shell_field.shell_layers)
shell_layers.nonelayer
Get solid field#
Get the solid field.
solid_field = stress[1]
Plot contour#
Plot the contour.
stress.plot_contour()
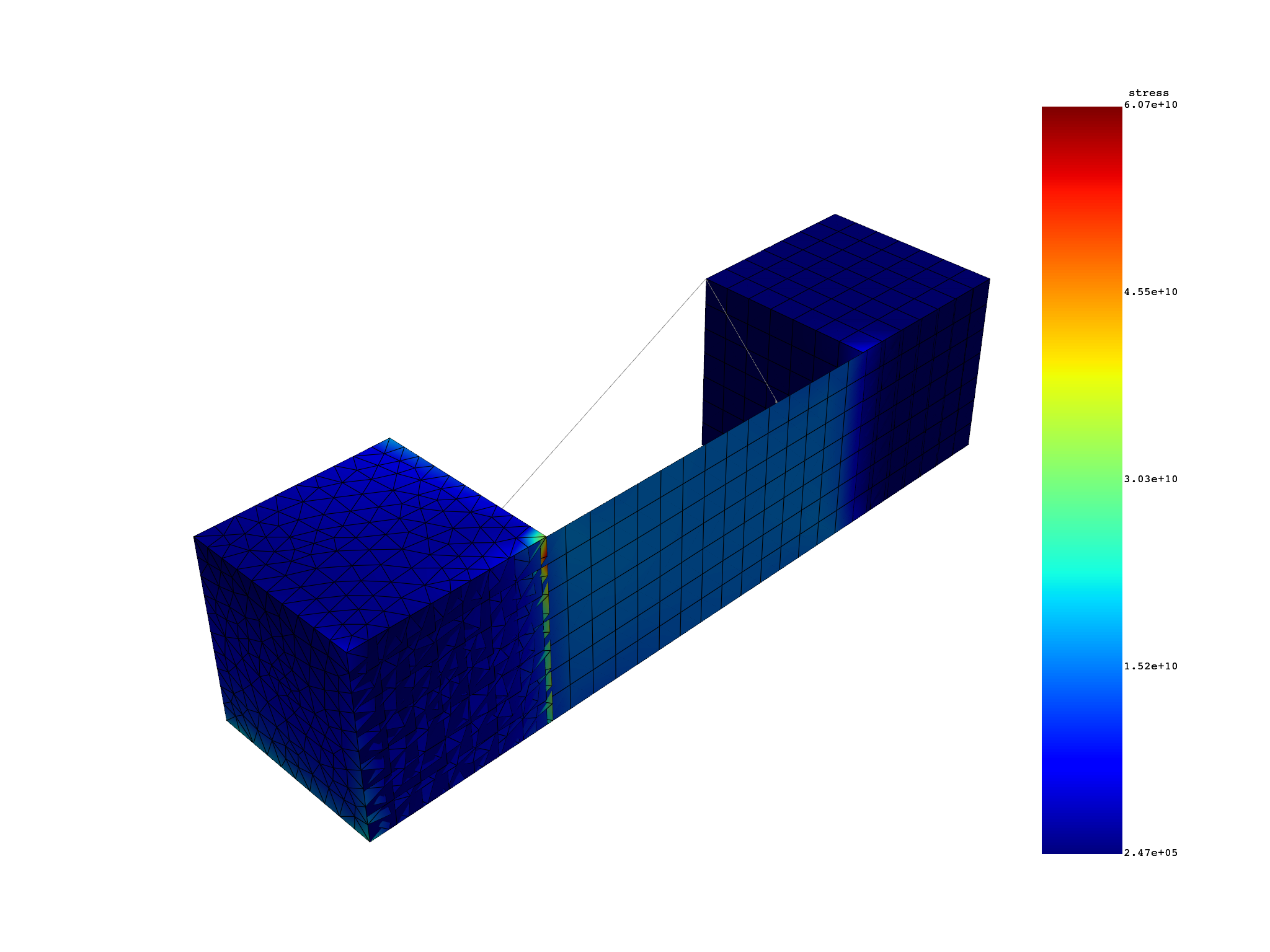
Get elastic strain result#
Get an elastic strain result.
elastic_strain_result = solution.elastic_strain()
elastic_strain = elastic_strain_result.tensor
Check number of fields#
Check the number of shell and solid elements in distinct fields.
print(elastic_strain.num_fields)
2
If the result file contains results, you can use this method to get the elastic strain result.
print(solution.plastic_strain())
Tensor object.
Object properties:
- location : Nodal
Plastic strain object.
You can also use this method to get the temperature result.
print(solution.structural_temperature())
Scalar object.
Object properties:
- location : Nodal
Structural temperature object.
Total running time of the script: (0 minutes 1.301 seconds)